Debugging in Script Engine
Transforming data using script languages might contains syntax errors or logical errors. To find out what happens in the script at any point in time, you can log the information using Script.log ()
, which can be further used for future reference and debugging. The script.log()
is a function in Script Engine that can be used to log any kind of variable or print any message to debug the custom scripts. It provides you an extra set of eyes that are constantly looking at the script and saving hours of debugging work.
Need for Logging
More information is always better. So the first step in detecting any problem is to increase the quantity of data you're logging. This enables you to observe everything going on both before and after an issue arises.
It offers complete tracing information.
If an error originates in a script, it is recorded.
Logging Limitation
There are certain limitations that you need to contemplated when performing script logging.
Limit Description | Limit Value | Notes |
---|---|---|
Maximum size of a single log | 1 MB | The objects that are logged and converted into string should not be greater than 1 MB |
Maximum numbers of log per batch | 1000 | Users are allowed to log 1000 values in a single batch. Each call to script.log() contributes to the log count.The example below will generate 1000 logs: for i in range(0, 1000): script.log("Logging", i) |
Syntax
Use this syntax to describe the event in the descriptive message. In the following example, we have used this function method to Log a message with no arguments. Only messages will be printed in logger Output.
script.log("This is a log")
script.log("Hi there", "This is a log")
// Will be logged as:
// Hi there This is a log
Logging a Variable
In most cases, you would want to log variables. The following example demonstrate variable logging.
:::codeblocktabs
var a = 10;
script.log(a);
var a = 10;
var b = 20.54;
var c = “Hello”
script.log(a, b, c);
// Will be logged as:
// 10 20.54 Hello
a = 10
script.log(a)
a = 10
b = 20.54
c = “Hello”
script.log(a, b, c)
# Will be logged as:
# 10 20.54 Hello
:::
Logging entire data from Pipeline
For debugging the entire pipeline, you can log the rows object provided in the function:
:::codeblocktabs
function process(rows, context) {
// some processing code here...
script.log(rows)
return rows
}
def process(rows, context):
# some processing code here...
script.log(rows)
return rows
:::
Output
[{Total Revenue=2533654, Units Sold=9925, Order ID=669165933, Sales Channel=Offline, Ship Date=2010-06-27T00:00:00.000+00:00, Item Type=Baby Food, Total Profit=951410.5, Total Cost=1582243.5, Unit Cost=159.42, Order Priority=H, Region=Australia and Oceania, Country=Tuvalu, Order Date=2010-05-28T00:00:00.000+00:00, Unit Price=255.28}, {Total Revenue=576782, Units Sold=2804, Order ID=963881480, Sales Channel=Online, Ship Date=2012-09-15T00:00:00.000+00:00, Item Type=Cereal, Total Profit=248406.36, Total Cost=328376.44, Unit Cost=117.11, Order Priority=C, Region=Central America and the Caribbean, Country=Grenada, Order Date=2012-08-22T00:00:00.000+00:00, Unit Price=205.7}, {Total Revenue=1158502, Units Sold=1779, Order ID=341417157, Sales Channel=Offline, Ship Date=2014-08-05T00:00:00.000+00:00, Item Type=Office Supplies, Total Profit=224598.75, Total Cost=933903.84, Unit Cost=524.96, Order Priority=L, Region=Europe, Country=Russia, Order Date=2014-02-05T00:00:00.000+00:00, Unit Price=651.21}]
Logging at Row Level
:::codeblocktabs
function process(rows, context) {
rows.forEach((row, rowNo) => {
// some processing code here...
script.log(rowNo, row)
})
return rows
}
def process(rows, context):
for row_now, row in enumerate(rows):
# some processing code here...
script.log(row_no, row)
return rows
:::
Output
0 {Total Revenue=2533654, Units Sold=9925, Order ID=669165933, Sales Channel=Offline, Ship Date=2010-06-27T00:00:00.000+00:00, Item Type=Baby Food, Total Profit=951410.5, Total Cost=1582243.5, Unit Cost=159.42, Order Priority=H, Region=Australia and Oceania, Country=Tuvalu, Order Date=2010-05-28T00:00:00.000+00:00, Unit Price=255.28
1 {Total Revenue=576782, Units Sold=2804, Order ID=963881480, Sales Channel=Online, Ship Date=2012-09-15T00:00:00.000+00:00, Item Type=Cereal, Total Profit=248406.36, Total Cost=328376.44, Unit Cost=117.11, Order Priority=C, Region=Central America and the Caribbean, Country=Grenada, Order Date=2012-08-22T00:00:00.000+00:00, Unit Price=205.7
2 {Total Revenue=1158502, Units Sold=1779, Order ID=341417157, Sales Channel=Offline, Ship Date=2014-08-05T00:00:00.000+00:00, Item Type=Office Supplies, Total Profit=224598.75, Total Cost=933903.84, Unit Cost=524.96, Order Priority=L, Region=Europe, Country=Russia, Order Date=2014-02-05T00:00:00.000+00:00, Unit Price=651.21}
Troubleshoot Incorrect Logging
While writing a script many times users log the variable class name instead of variable value.
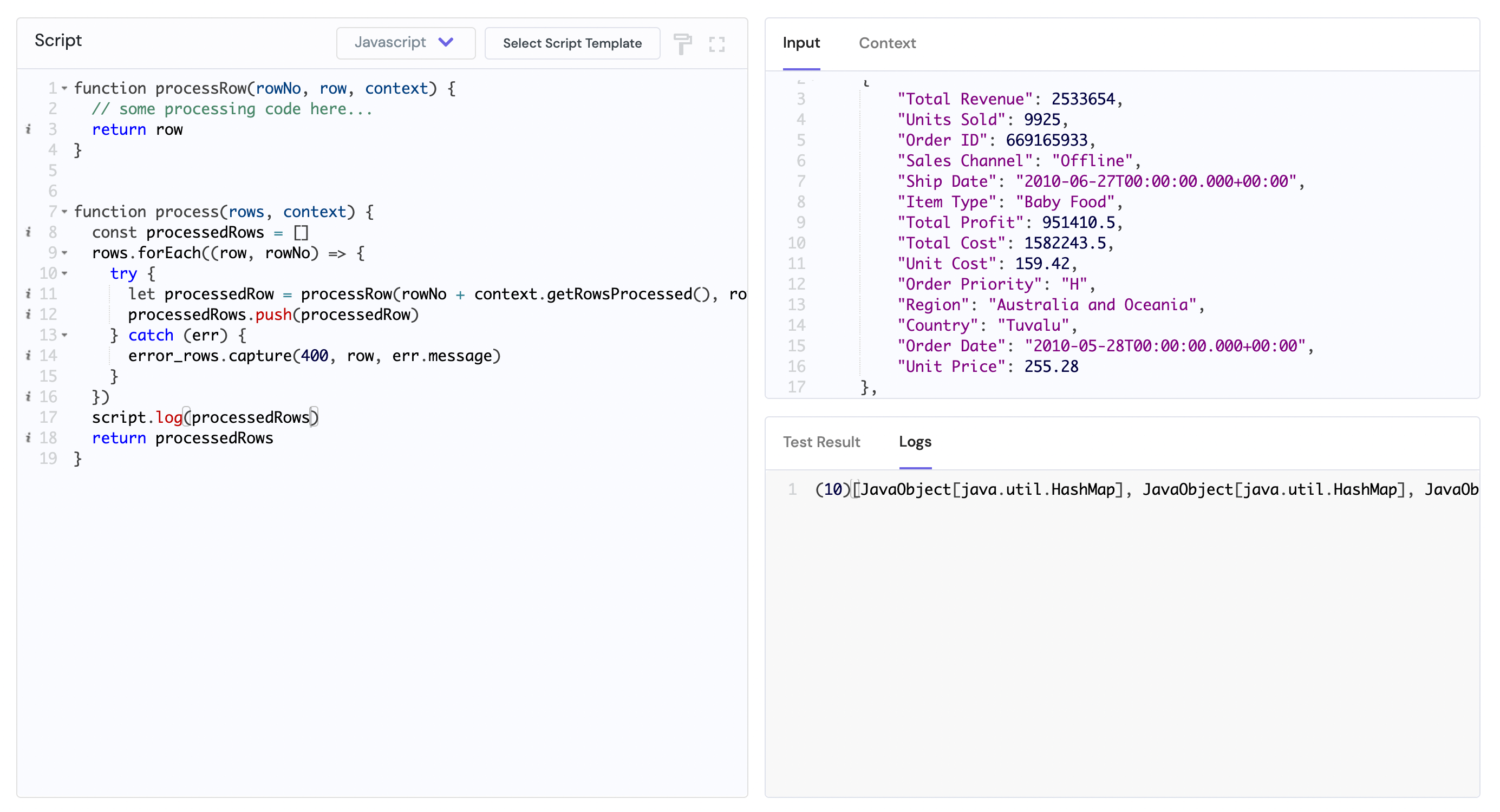
The following steps can be used to troubleshoot this
- Convert the variable to string before logging
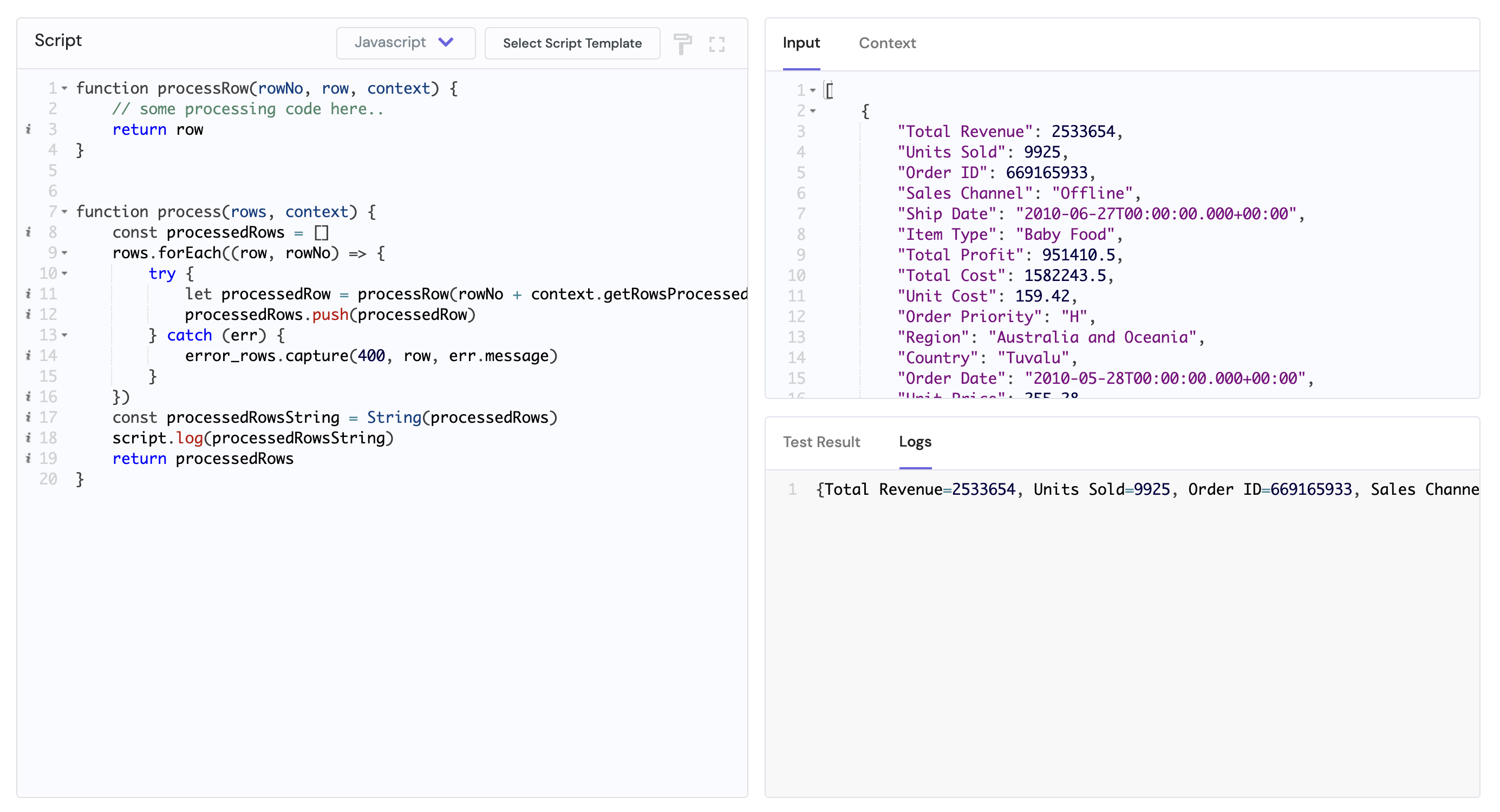
2. Log at element level by iterating if variable is an array
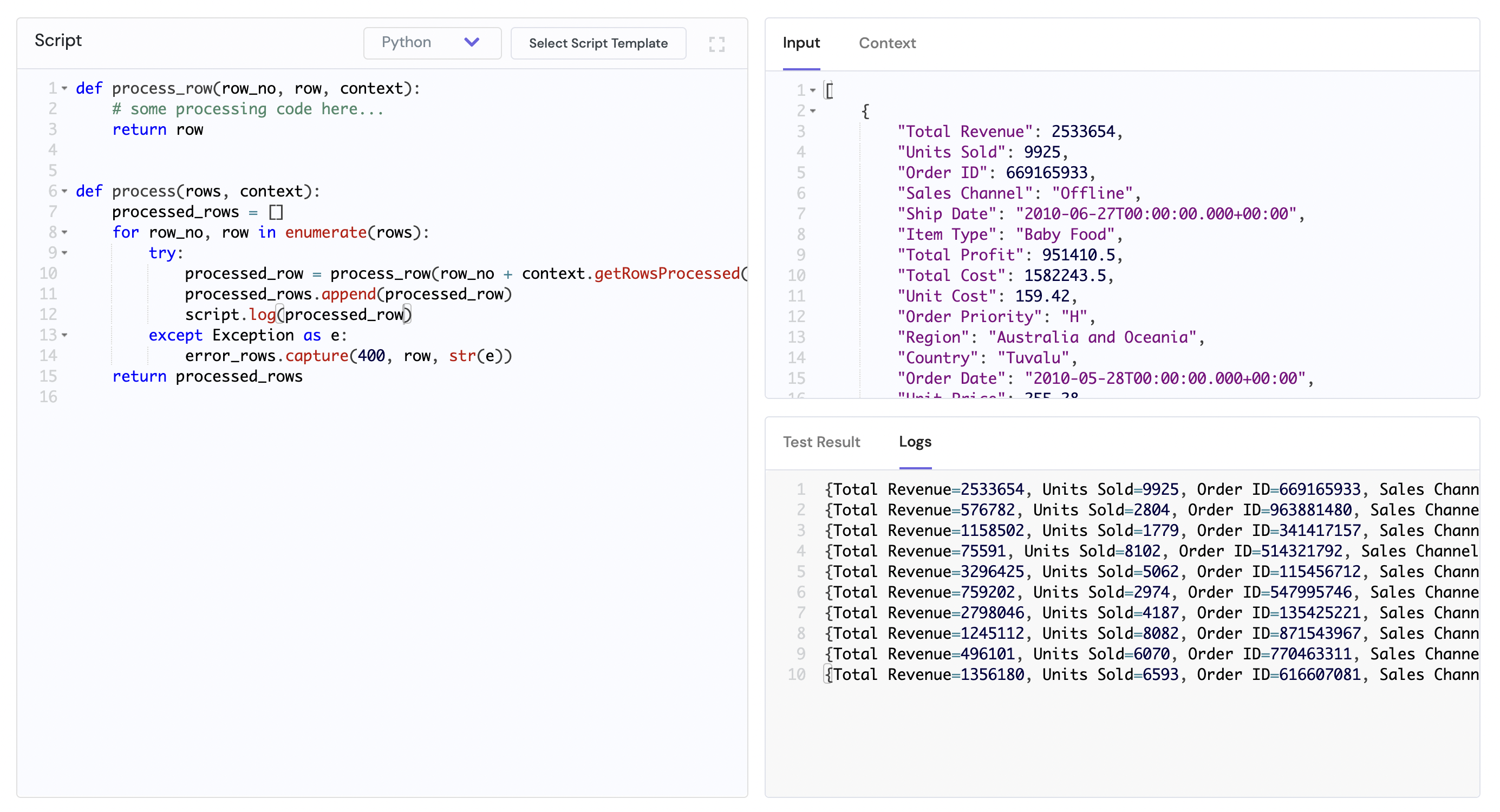
3. Use native java method to extract the required value from java variables
At times the values of the variables are appropriately logged, but the script does not function as intended. As seen below, when logging the value of Unit Price, they appear to be decimal values, but when using comparison operators on them, errors get occurred.
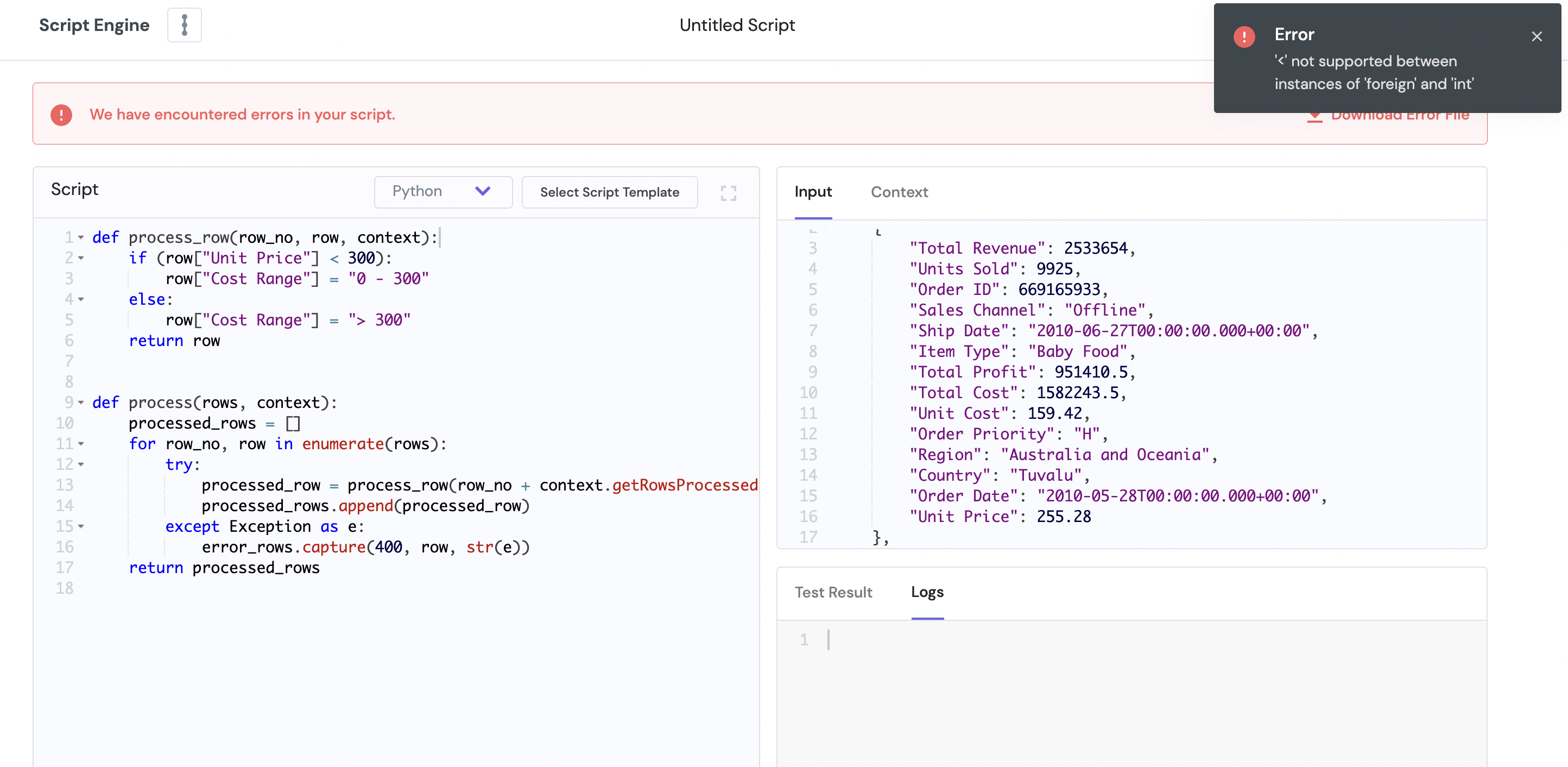
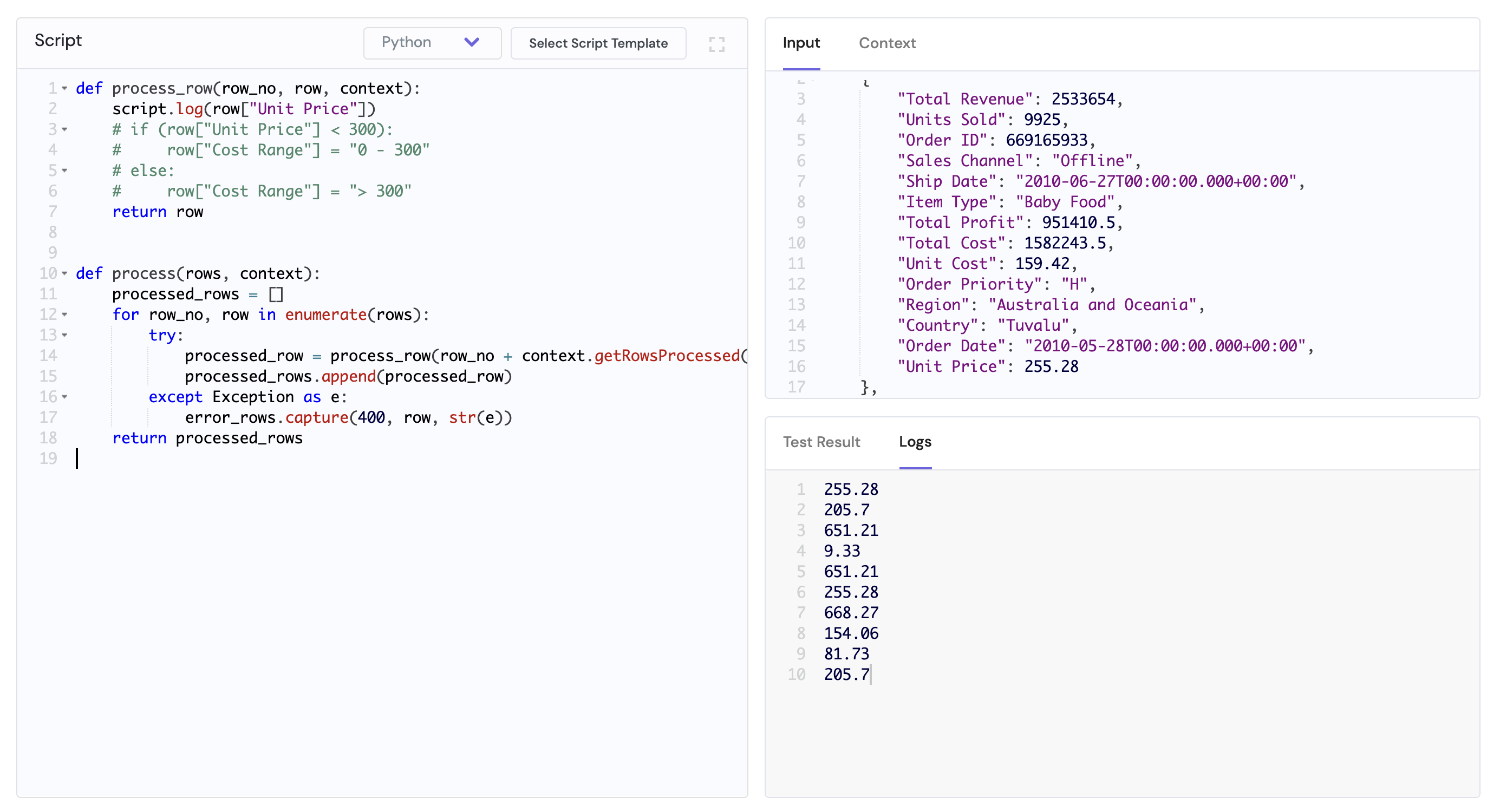
When you face this error, we request you to log the variables by converting them to String to check their actual datatypes:
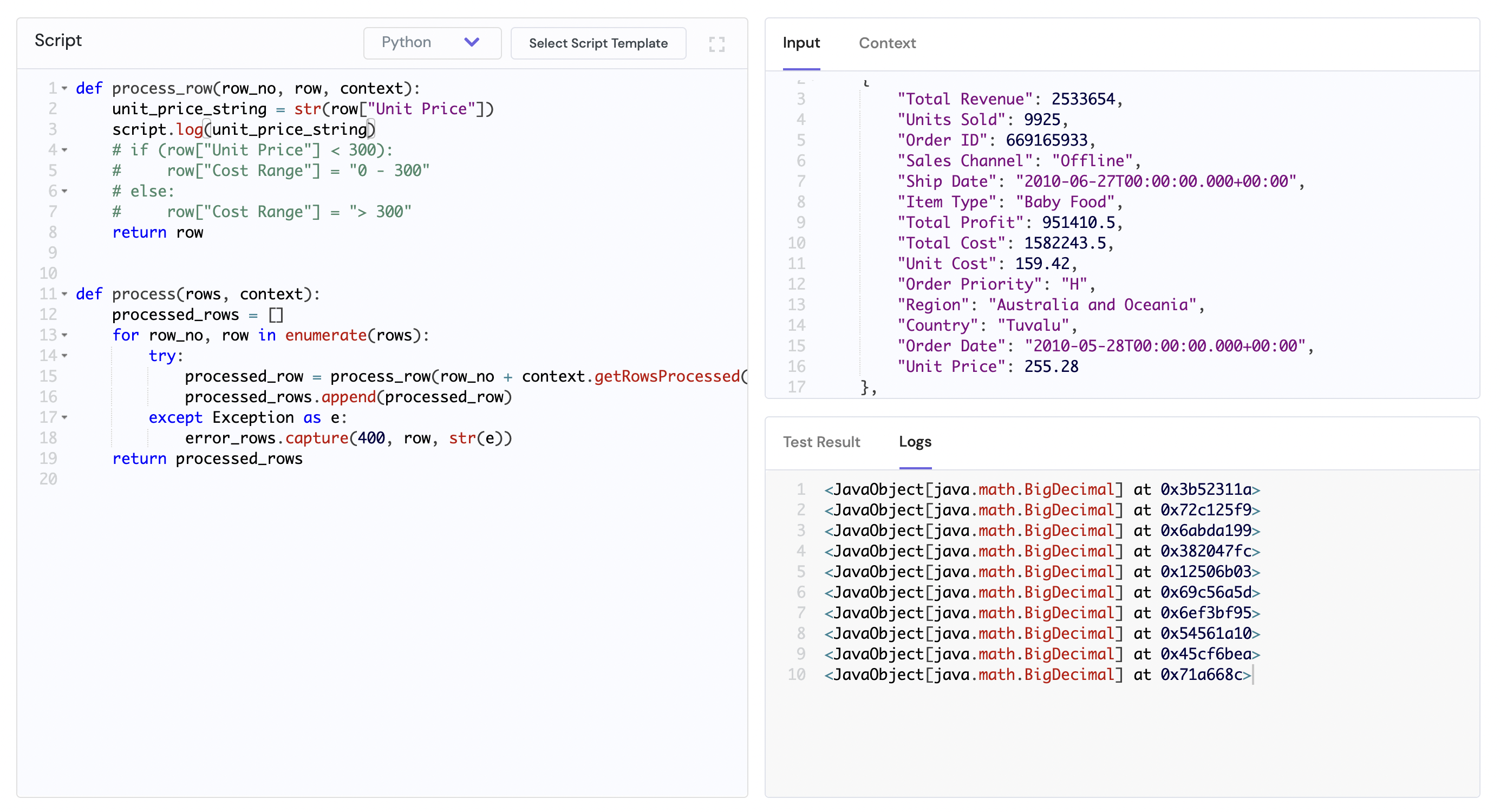
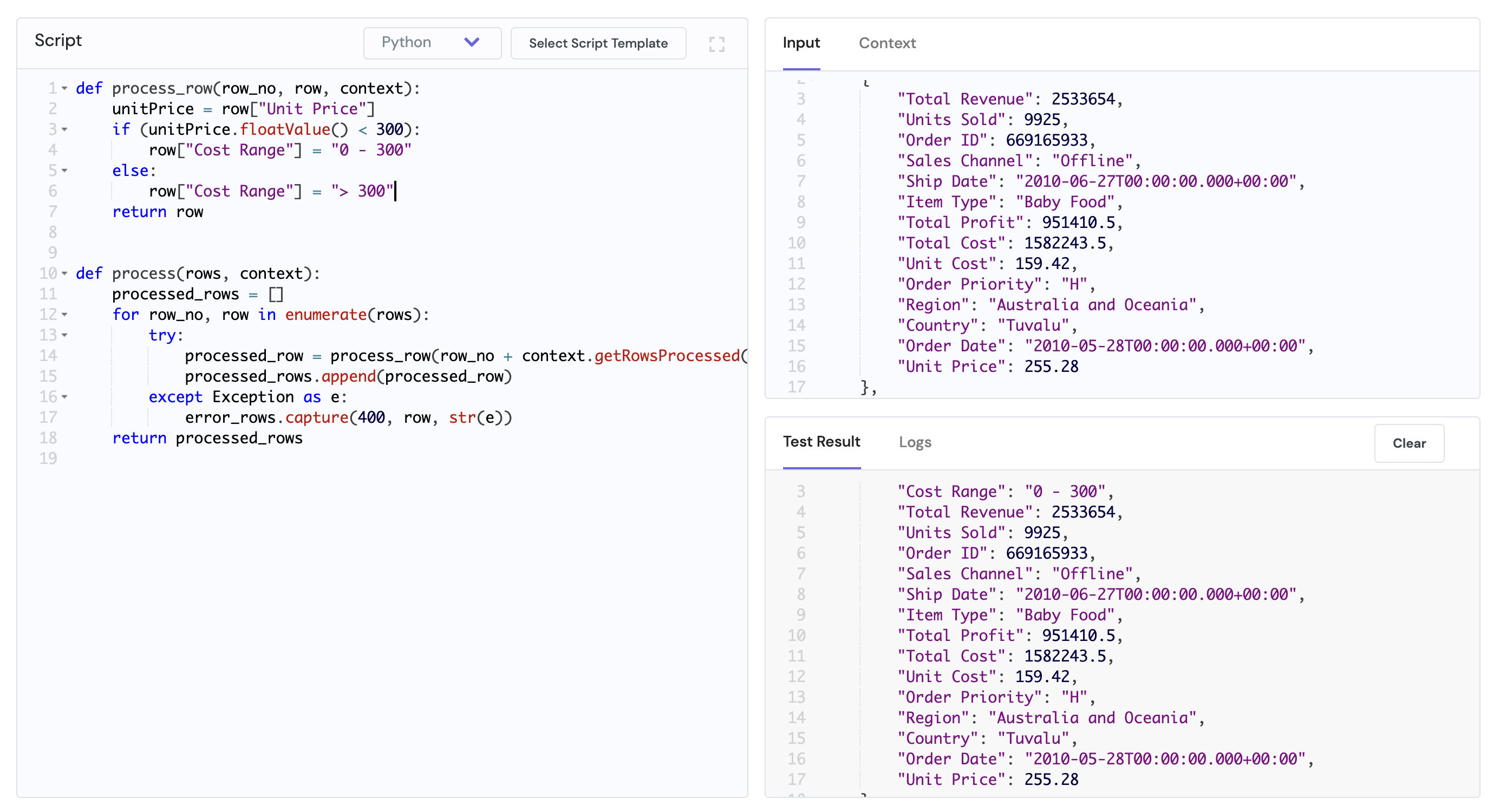
Any Question? 🤓
We are always an email away to help you resolve your queries. If you need any help, write to us at - 📧 support@boltic.io