REST API in PHP refers to rules for creating web services that allow communication between different systems. A RESTful API, also known as a RESTful web service, is an architectural style for developing web services based on representational state transfer (REST) technology. REST is an architectural style for building network-based software, which is used in the design of web services. One of the advantages of using RESTful APIs is that they can be easily integrated with other systems and technologies.
With REST, you can retrieve data from a web server using simple HTTP requests, making connecting your PHP applications with other services and systems possible. This blog will explore REST APIs and the rules for building RESTful APIs. Additionally, we'll cover the main criteria for inclusion in our list of the best PHP REST API frameworks and walk through the process of building a simple REST API in PHP. Lastly, we'll compare some of the best PHP frameworks for REST APIs, including Laravel, Lumen, Guzzle, and Epiphany.
What is REST API?

REST stands for Representational State Transfer, an architectural style for designing network-based software. RESTful APIs, also known as RESTful web services, are web services that implement this architectural style. REST is based on the principles of the HTTP protocol, which is used for communication between different systems on the internet. With REST, you can use simple HTTP requests to retrieve or manipulate data on a web server. For example, you can use a GET request to retrieve data from a server, a POST request to create a new resource on the server, a PUT request to update an existing resource, and a DELETE request to delete a resource.
One of the benefits of using REST is that it makes it possible to integrate your PHP application with other systems and services. RESTful APIs are platform-independent, meaning you can use them with any programming language. They are also language-independent, meaning you can use them with any language. In RESTful APIs, resources are represented by URIs, and the state of these resources can be manipulated using the HTTP methods. For example, you can use a GET request to retrieve help from a server, a POST request to create a new resource, a PUT request to update an existing resource, and a DELETE request to delete a resource.
Overall, REST is a simple and flexible architecture that is easy to use and provides many benefits for building network-based software. With REST, you can create web services that can be easily integrated with other systems and technologies, which makes it an excellent choice for building REST APIs in PHP.
What are the rules for Building REST APIs?
REST APIs have a set of constraints that define how they should be built and used. These constraints are called the rules for making RESTful APIs and are also known as design constraints.

The main design constraints for building RESTful APIs are:
- Uniform Interface: The consistent interface constraint states that all resources should be accessed constantly using the same methods. This consistent interface makes it possible to understand how to access and manipulate resources, regardless of the underlying technology.
- Client-Server: The client-server constraint states that the client and server should be separate components and that the server should not know anything about the client. This constraint allows the client and server to be developed independently and allows replacing one component without affecting the other.
- Stateless: The stateless constraint states that each request from a client should contain all the information necessary to understand the request and that the server should not have to store any client context between requests. This constraint makes it possible to scale the server by adding additional servers without worrying about maintaining the client state.
- Cacheable: The cacheable constraint states that responses from the server should include information about whether they can be cached and for how long. This constraint makes it possible to cache responses, reducing the server load and improving the API's performance.
- Layered System: The layered system constraint states that the API should be designed as a layered system, with each layer adding additional functionality to the API. This constraint makes it possible to understand the API and to add or remove functionality as needed.
- Code on Demand: The code on demand constraint states that the API may include the ability to download code to be executed on the client. This constraint allows for more advanced functionality to be included in the API without relying on the client to provide it.
By following these design constraints, you can ensure that your RESTful API is easy to use, understand, and maintain. Additionally, following these constraints will help ensure your API is scalable, flexible, and reliable.
Main criteria for inclusion in this List
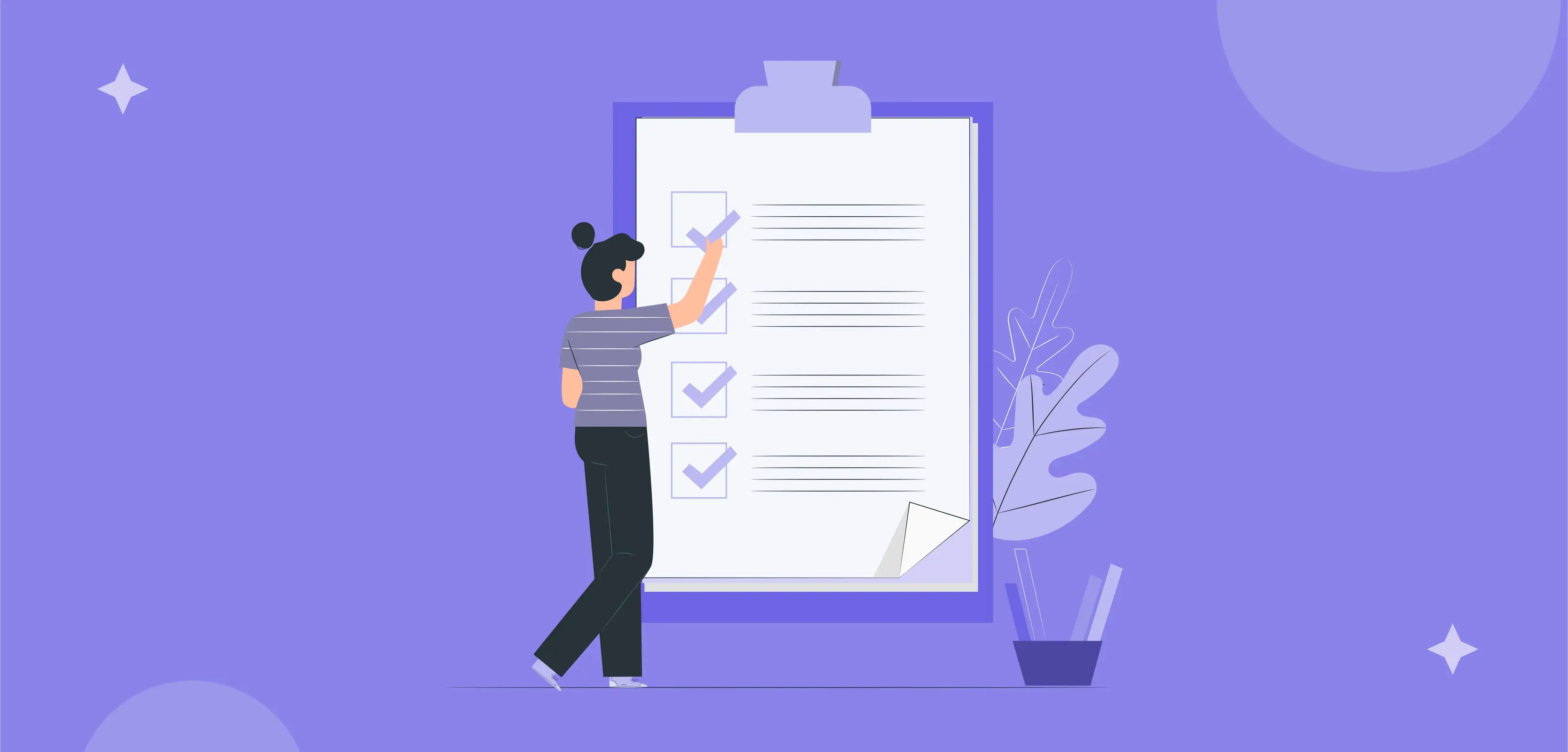
Several factors should be considered when evaluating the best PHP REST API frameworks. The following are the main criteria for inclusion in this list:
- API Focus: The framework should be designed specifically for building REST APIs and provide the tools and features to make it easy to build, test, and maintain a REST API.
- Gentle Learning Curve: The framework should be easy to learn and use, with a gentle learning curve that makes it accessible to developers of all skill levels.
- Active Community: The framework should have an active and supportive community, providing resources, tutorials, and user support. This framework helps ensure that the framework is well-maintained and that issues and bugs are quickly addressed.
By considering these criteria, you can be confident that the PHP REST API frameworks you choose will best fit your needs and help ensure your REST API is successful.
How to build a simple REST API in PHP?
Building a REST API in PHP is relatively straightforward and can be done with just a few lines of code. You'll need a basic understanding of PHP and its syntax and some experience with server-side web development to get started.
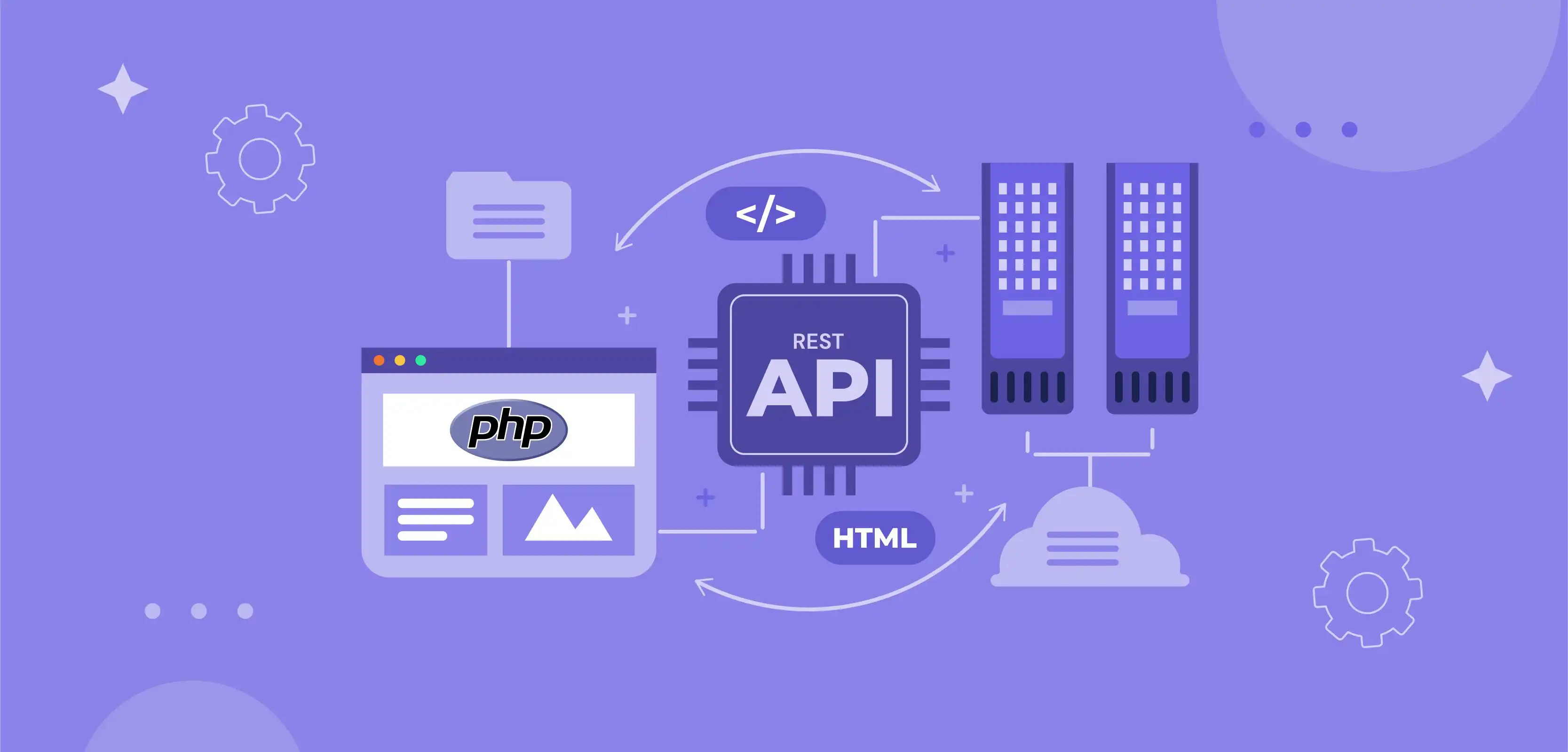
Here's a simple example of how you might build a REST API in PHP:
- First, set up a new PHP project. You can do this using a framework like Laravel or starting from scratch with a basic PHP installation.
- Next, decide on the endpoints you want to create for your REST API. These endpoints will define the functionality of your API, and I will determine what data your API can return to clients.
- Create a new PHP file for each endpoint, and use PHP functions to retrieve data from a database or other data source. For example, you might use a function like get_data() to retrieve data from a MySQL database.
- Use the header() function to set the HTTP response code for each endpoint and return the data as a JSON string using the json_encode() function.
- Test your REST API using a tool like Postman or sending HTTP requests directly from a client-side script.
Following these steps, you can build a simple REST API in PHP to return data to clients over HTTP. Of course, this is just a simple example, and there is much more that you can do with a REST API in PHP. The best way to learn more is to experiment with different frameworks and techniques and to get hands-on experience building REST APIs.
What are the Best PHP REST API Frameworks?
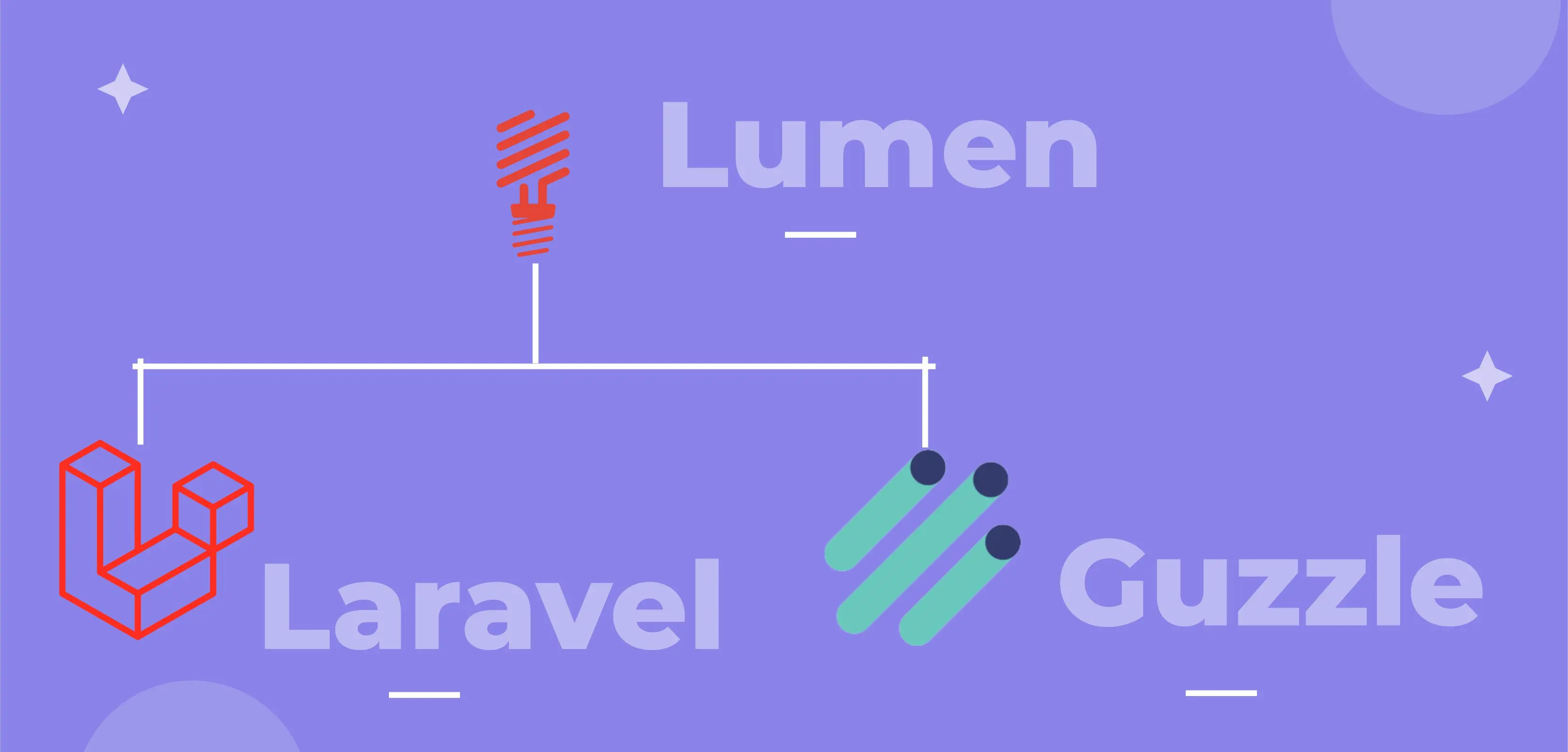
There are several different frameworks and modules available for PHP developers to create REST APIs; some of the most popular include:
- Laravel: Laravel is a comprehensive PHP framework with tools for building REST APIs and other features. It is well-documented and has a large community of users and developers.
- Lumen: Lumen is a lightweight version of Laravel specifically designed to build REST APIs. It is fast, simple to use, and comes with various tools for working with HTTP requests and responses.
- Guzzle: Guzzle is a PHP library working with HTTP requests and responses. It can be used for building REST APIs and provides a simple, easy-to-use interface for working with HTTP data.
- Epiphany: Epiphany is a minimalistic PHP framework designed to build REST APIs. It is simple to use and provides various tools for handling HTTP requests and responses, routing and handling errors.
Each of these frameworks has its strengths and weaknesses, and the best choice for your project will depend on your specific needs and requirements. When deciding, it is worth evaluating each framework carefully and considering factors such as ease of use, performance, and community support.
Summary: Best PHP Frameworks for REST APIs
There are numerous frameworks available for PHP when developing REST APIs; this section compares some of the most popular options based on key features and characteristics.
{{phpframework="/components"}}
As you can see from the table, Laravel and Lumen are two of the most popular options, offering strong API focus, gentle learning curves, and active communities. On the other hand, Guzzle is a more straightforward library that may be easier to start with, but it does not offer as much support and community resources as Laravel or Lumen. Finally, Epiphany offers a minimalistic approach to building REST APIs, focusing on simplicity and ease of use, but has a smaller community. Ultimately, the best choice for your project will depend on your specific needs and requirements. It's essential to evaluate each option carefully and consider factors such as performance, ease of use, and community support.
Scaling REST APIs in PHP for high traffic & performance
Scaling REST APIs in PHP for high traffic and performance is critical, especially when the application is expected to handle multiple user requests simultaneously. Scaling aims to ensure that the API can accommodate the growing demand while maintaining an acceptable level of performance and reliability. Here are some best practices to help you scale REST APIs in PHP:
1. Use a caching mechanism:
Caching is a technique that helps reduce the server load by temporarily storing frequently accessed data. This technique can significantly improve the performance of your REST API, especially during periods of high traffic. You can use caching mechanisms such as Memcached, APC, and Redis to cache the results of database queries, API responses, and other frequently accessed data.
2. Load balancing:
Load balancing distributes incoming traffic among multiple servers to ensure no single server becomes overloaded. Load balancing can help to prevent server downtime, slow response times, and other performance issues that can arise when too many requests are sent to a single server. You can use hardware or software load balancers to distribute traffic among multiple servers.
3. Database optimisation:
Database optimisation is crucial when scaling REST APIs in PHP. A poorly optimised database can cause slow response times, timeouts, and other performance issues. You can optimise your database by using indexing, partitioning, and sharing techniques, as well as by reducing the number of database queries and optimising database query performance.
4. Use a Content Delivery Network (CDN):
A CDN is a network of servers located in multiple locations. When you use a CDN, your API data is cached and served from a server closest to the user, which can help to reduce latency and improve performance. This server network is essential for global REST APIs, which aid users from multiple locations.
5. Monitor performance and usage:
Monitoring performance and usage are essential for identifying performance bottlenecks and other issues when scaling REST APIs in PHP. You can use tools such as New Relic, LogRocket, and Amazon CloudWatch to monitor performance, track response times, and identify issues that must be addressed. By following these best practices, you can scale your REST APIs in PHP to handle high traffic and maintain acceptable performance and reliability. It is also essential to continuously monitor and optimise your REST APIs, especially as your application grows and evolves.
Advantages of using REST APIs in PHP
REST APIs have become famous for building web services due to their simplicity, flexibility, and scalability. This section will discuss some key benefits of using REST APIs in PHP.
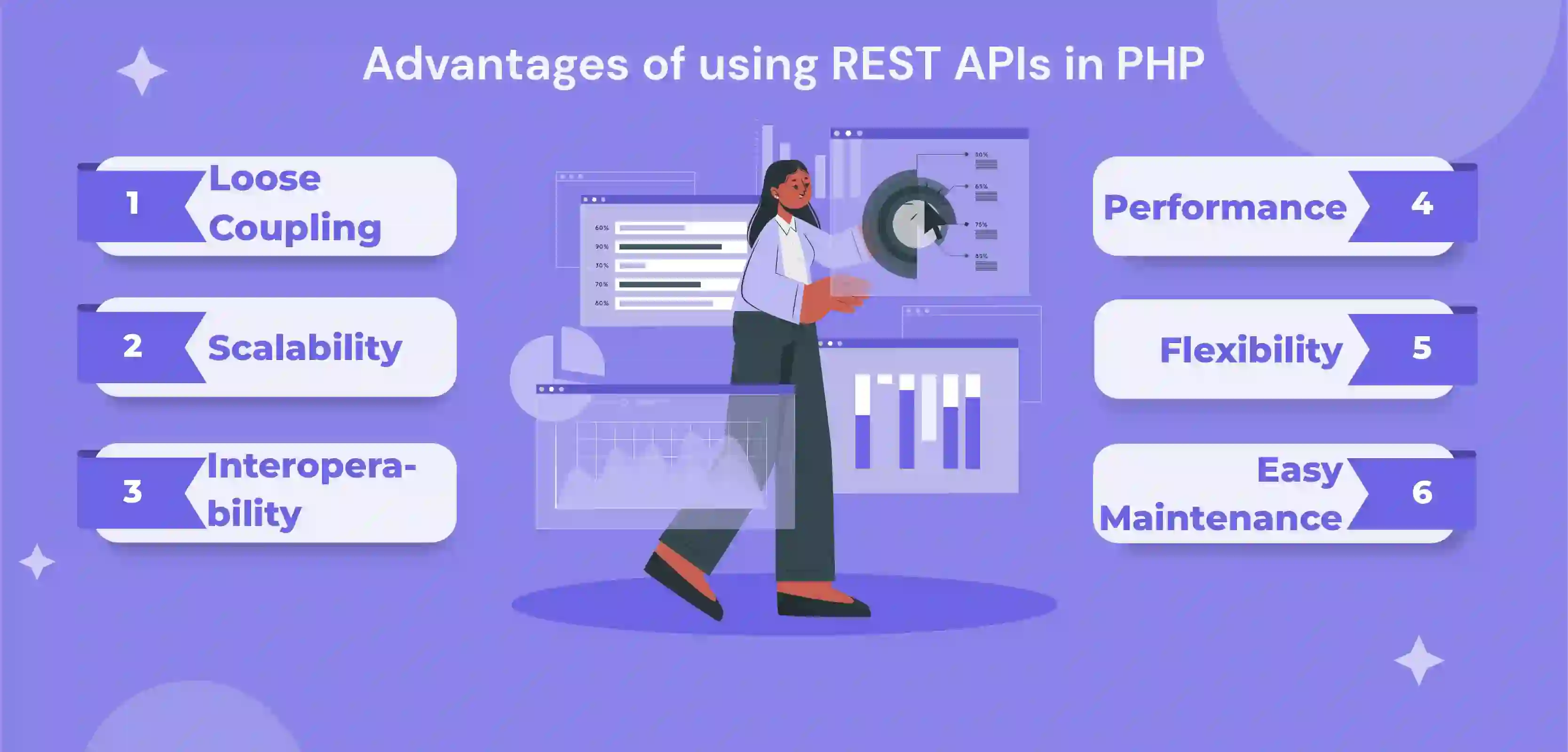
1. Loose Coupling:
REST APIs promote loose coupling between the client and server, which means that changes made on either side will have minimal impact on the other. This method is because REST APIs rely on HTTP for communication, a well-established and widely adopted protocol.
2. Scalability:
REST APIs are easy to scale, as they don't have specific requirements for server-side infrastructure. As your application grows, you can easily add new resources and services to meet the increasing demand.
3. Interoperability:
REST APIs can be easily integrated with other systems, as they are based on standard HTTP methods and data formats. This integration makes connecting different techniques, such as mobile apps, web applications, and backend services, easy without worrying about compatibility issues.
4. Performance:
REST APIs are lightweight and fast, as they don't have to deal with complex data structures or communication protocols. This nature of REST APIs makes them ideal for building high-performance web services that handle large amounts of traffic and data.
5. Flexibility:
REST APIs are highly flexible and can be used for many applications and use cases. The flexibility can build REST APIs to meet specific needs, whether the app or a complex enterprise system.
6. Easy Maintenance:
REST APIs are easy to maintain, based on standard protocols and data formats. So, developers can easily understand and modify the code, even if this is their first time working with the API.
7. Language Independent:
REST APIs can be built using a variety of programming languages, including PHP. You can choose the language that best fits your needs and your team's expertise. So, the advantages of using REST APIs in PHP include loose coupling, scalability, interoperability, performance, flexibility, easy maintenance, and language independence. These benefits make REST API attractive for building web services that handle various requirements and use cases.
Use cases & real-world examples of REST APIs in PHP.
REST APIs have been widely adopted as a standard for building web-based applications due to their simplicity, flexibility, and scalability. Many real-world examples of REST APIs in PHP demonstrate this technology's power and versatility. Some of the most common use cases for REST APIs include the following:
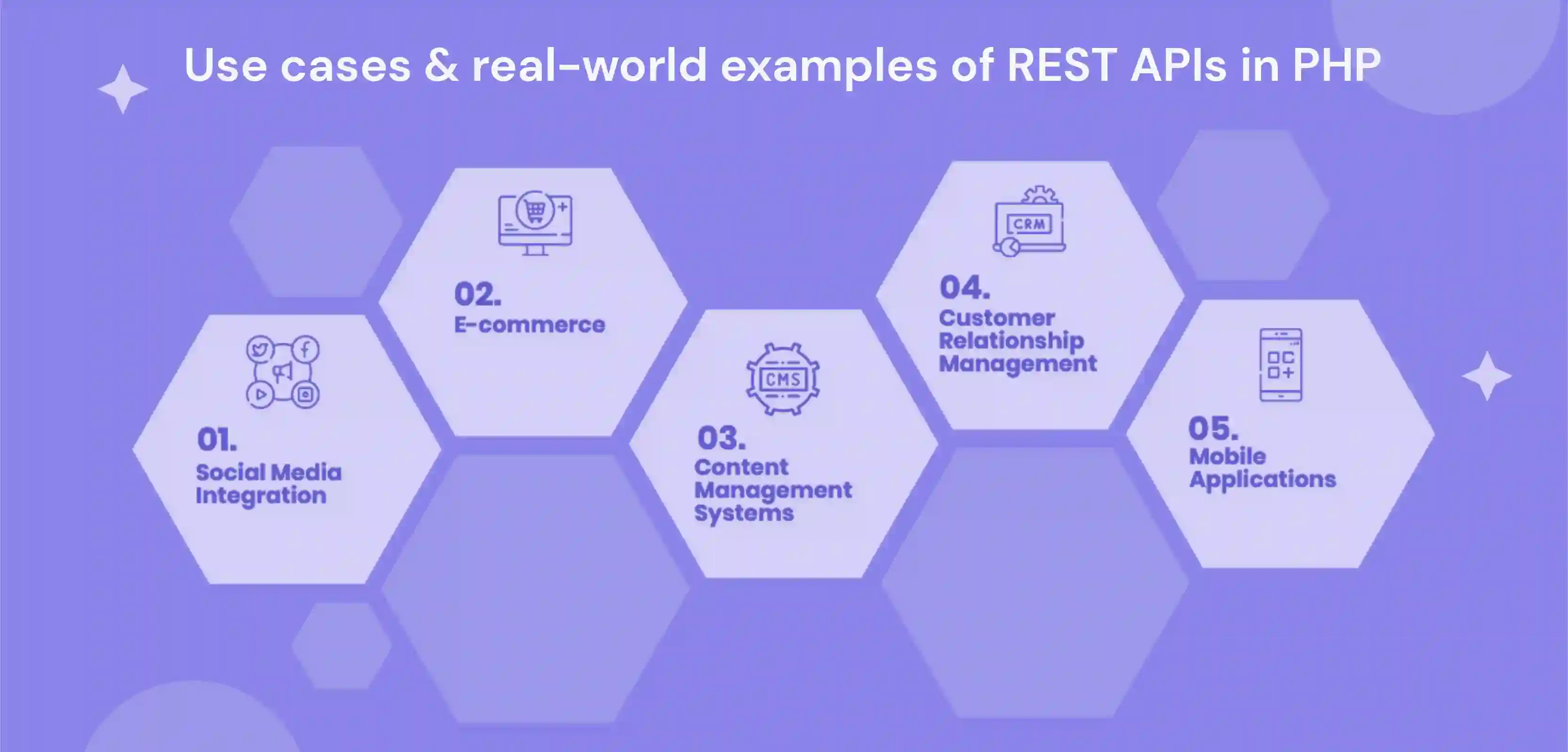
1) Social Media Integration:
REST APIs can integrate social media platforms like Facebook and Twitter with your PHP-based website. This integration allows you to access and display user data from these platforms on your website, such as friend lists, statuses, and more.
2) E-commerce:
REST APIs can integrate e-commerce platforms like Magento and Shopify with your PHP-based website. This method allows you to access and display product catalogues, customer data, and order information from these platforms on your website.
3) Content Management Systems (CMS):
REST APIs can integrate content management systems like WordPress and Drupal with your PHP-based website. This integration method allows you to access and display blog posts, pages, and other content from these platforms on your website.
4) Customer Relationship Management (CRM):
REST APIs can integrate customer relationships management systems like Salesforce and Hubspot with your PHP-based website. This algorithm can allow you to access and display customer data and lead information from these platforms on your website.
5) Mobile Applications:
REST APIs can power mobile applications, bridging the mobile application and your PHP-based website. This system allows you to access and display data from your website within your mobile application, such as products, customer data, and more. In conclusion, REST APIs have many use cases and real-world examples in PHP, making them a versatile and powerful technology for building web-based applications.
Whether you want to integrate social media platforms, e-commerce platforms, content management systems, customer relationship management systems, or mobile applications, REST APIs are an excellent solution for accessing and displaying data from these platforms in your PHP-based website.
Integrating REST APIs in PHP with other technologies: Databases & Front-End Frameworks
Integrating REST APIs in PHP with other technologies, such as databases and front-end frameworks, is critical to building modern web applications. This integration allows different systems to communicate and exchange data, creating complex and dynamic applications that meet user requirements. When integrating REST APIs in PHP with databases, it is essential to consider the type of data exchanged between the two systems. For example, suppose the REST API will retrieve data from a database. In that case, it may be necessary to implement an Object-Relational Mapping (ORM) layer that can translate the data from the database into a more appropriate format for use in the API.
Similarly, the REST API will store data in a database. In that case, it may be necessary to implement a data validation layer to ensure that stored data is consistent and meets the database schema requirements. When integrating REST APIs in PHP with front-end frameworks, it is vital to consider the type of data displayed in the user interface. For example, the REST API will use several display tables. In that case, it may be necessary to implement a data grid component to display the data in a tabular format. Similarly, suppose the REST API will be used to express a chart; in that case, it may be necessary to implement a charting component to display the data in a graphical format.
Another important consideration when integrating REST APIs in PHP with other technologies is security. Implementing appropriate security measures to protect sensitive data, such as encryption and authentication, is important. For example, it may be necessary to implement an authentication layer to validate the user's identity to make the API request and to implement encryption to protect sensitive data transmitted between the API and other systems.
So, integrating REST APIs in PHP with other technologies, such as databases and front-end frameworks, is critical to building modern web applications. By considering the type of data that will be exchanged, implementing appropriate security measures, and choosing the right tools and technologies, developers can build robust and scalable REST APIs that meet a wide range of user requirements.
Common pitfalls to avoid when Building REST APIs
Building REST APIs can be challenging, and it's essential to understand the common pitfalls to avoid them when developing your API. Here are some of the most common pitfalls to avoid. Not following REST principles: One of the biggest pitfalls is not following REST principles such as uniform interface, client-server architecture, statelessness, and cache ability. Adhering to these principles is essential for building a robust and scalable API.
1. Inconsistent API endpoints:
Another common pitfall is unreliable API endpoints. Ensuring that all API endpoints follow a consistent naming convention and structure is essential. This practice makes it easier for developers to understand and work with the API.
2. Ignoring error handling:
Error handling is an important aspect of API development, but it's often overlooked. You must ensure that your API provides clear and concise error messages so developers can understand what happened and quickly resolve the issue.
3. Undocumented API:
Undocumented APIs can cause confusion and make it difficult for developers to work with the API. It's important to provide clear and concise documentation, including examples and explanations, so developers can understand how to use the API effectively. Not using the correct HTTP methods: HTTP methods such as GET, POST, PUT, and DELETE have specific meanings, and it's essential to use them correctly. Using these methods can result in unexpected behaviour and make it easier for developers to work with the API.
4. Lack of security:
Security is critical for APIs, and it's important to implement measures such as encryption and authentication to prevent unauthorised access. Neglecting safety can lead to severe consequences, including data breaches and loss of sensitive information. Thus, building REST APIs can be challenging, but understanding the common pitfalls and taking the necessary measures to avoid them can help ensure that your API is robust, scalable, and secure. By following the best practices and guidelines, you can create a high-quality API that provides a seamless experience for developers.
Debugging & Testing REST APIs in PHP
Debugging and testing REST APIs in PHP is a crucial step in development. The goal of testing and debugging is to identify any errors or bugs in the code that may impact the functionality of the API. This step is critical to ensure the API's reliability and security and avoid any unexpected behaviour that may affect the end-users. Several tools and methods can be used to test and debug REST APIs in PHP. Let's take a look at some of the best practices:
Automated testing involves writing test cases for each API endpoint to ensure it works as expected. This testing can be done using frameworks like PHPUnit or Behat, allowing you to write and test cases automatically. Automated testing helps to catch bugs early in the development process and saves time and effort compared to manual testing.
1) Manual testing:
Although automated testing is helpful testing, the API is important API to ensure it works as expected. This test can be done by sending HTTP requests to the API endpoints and checking the response. Tools like Postman or cURL can send these requests and check the answers.
2) Logging:
Logging is a helpful tool for debugging and troubleshooting REST APIs. By logging the requests and responses, you can quickly identify any errors or issues with the API. Using proper logging tools and techniques is important to ensure the logs are easy to read and understand.
3) You are debugging tools:
Tools like Xdebug to debug PHP code by stepping through the code, setting breakpoints, and inspecting variables. These tools can be beneficial when you're trying to identify an issue with the API. Code reviews are essential to the development process and can be especially helpful in debugging and testing REST APIs. Having other developers review, your code can catch bugs and errors you may have missed.
Testing and debugging are critical steps in developing REST APIs in PHP. By following best practices, such as automated testing, manual testing, logging, debugging tools, and code reviews, you can ensure the reliability and security of your API and avoid any unexpected behaviour.
Best practices for designing REST APIs
When designing REST APIs in PHP, several best practices can help ensure your APIs are well-structured, scalable, and maintainable. Some of these best practices include:

- Using HTTP Verbs Consistently: HTTP verbs like GET, POST, and PUT should be used consistently to perform their intended functions, making it easier for developers to understand and use your APIs.
- Naming Resources Appropriately: Resource names should be descriptive and meaningful, making it easier for developers to understand the relationships between resources.
- Using Proper HTTP Status Codes: HTTP status codes indicate the success or failure of an API request. Ensure you're using the correct status codes for each scenario so developers can understand what's happening with their requests.
- Versioning Your APIs: As your APIs evolve, it's important to version them so that existing applications can continue to work even as you make changes. Consider using version numbers in your API URLs to make this process easier.
- Implementing Error Handling: Error handling is an important aspect of building REST APIs. You should have a transparent process for handling errors and returning meaningful error messages to developers so they can quickly understand what went wrong and how to fix it.
- Documenting Your APIs: Good documentation is critical to ensuring developers can effectively use your APIs. You should have clear and thorough documentation that explains how to use the API, what to anticipate from the answer, and how to spot errors.
- Using Pagination: If your APIs return many results, consider using pagination to break them into smaller chunks, making it easier for developers to work with your APIs and reducing the load on your servers.
- Caching Responses: Caching API responses can help improve performance and reduce server load. Consider using HTTP cache headers to specify how answers should be cached and for how long.
By following these best practices, you can help ensure that your REST APIs are well-designed and effective for developers.
Security Considerations for REST APIs in PHP
Security should be a top priority when building REST APIs in PHP because APIs are often used to transfer sensitive information, such as user credentials and personal data. As a result, it's essential to have proper security measures in place to protect this information and prevent unauthorised access. Here are some of the most important security considerations when building REST APIs in PHP.
1. Authentication & Authorisation
Authentication is the process of verifying the identity of a user, while authorisation is the process of determining what resources a user is allowed to access. When building a REST API, it's important to implement proper authentication and authorization mechanisms to ensure that only authorised users can access the API. This authorisation can be achieved using various methods, including basic authentication, token-based authentication, and OAuth.
2. Encryption
Encryption is the process of encoding data so unauthorised users cannot read or understand it. Encryption is important when building a REST API to protect sensitive information, such as passwords, financial data, and personal information. This encryption can be achieved by using SSL or TLS encryption, which encrypts data in transit between the client and server.
3. Input Validation
Input validation checks user-supplied data to ensure it meets the required format and type. When building a REST API, validating user inputs to prevent malicious attacks, such as SQL injections and cross-site scripting (XSS), is important. This validation can be achieved using input validation libraries and functions built into the PHP language.
4. Rate Limiting
Rate limiting limits a user's requests to an API within a specified period. When building a REST API, it's important to implement rate limiting to prevent excessive use of resources and potential attacks, such as denial-of-service (DoS) attacks. This limiting can be achieved using rate-limiting libraries and functions built into the PHP language.
5. Logging
Logging is the process of recording events and information about the API in a log file. When building a REST API, it's important to implement proper logging to track and diagnose security incidents, such as unauthorised access and data breaches. This process can be achieved using logging libraries and functions built into the PHP language. In conclusion, security is essential when building REST APIs in PHP. You can ensure that your API is secure and protects sensitive information from unauthorised access and attacks by implementing proper authentication and authorisation mechanisms, encryption, input validation, rate limiting, and logging.
Conclusion
REST APIs are crucial in today's technology landscape, enabling communication between systems and devices. With PHP being one of the most popular server-side scripting languages, it's no surprise that many robust frameworks are available to help developers build high-quality REST APIs. In this blog, we've covered the basics of REST APIs, including what they are, the rules for building them, and how to build a simple REST API in PHP. We've also explored some of the best PHP REST API frameworks and discussed key topics such as scaling, security, debugging, testing, and integration.
By following the best practices outlined in this blog and considering the challenges and limitations of REST APIs, developers can build reliable, scalable, and secure REST APIs that meet the needs of their users. Whether you're a seasoned PHP developer or just starting, this guide provides a comprehensive overview of REST APIs in PHP and will help you get started on your next project.
drives valuable insights
Organize your big data operations with a free forever plan
An agentic platform revolutionizing workflow management and automation through AI-driven solutions. It enables seamless tool integration, real-time decision-making, and enhanced productivity
Here’s what we do in the meeting:
- Experience Boltic's features firsthand.
- Learn how to automate your data workflows.
- Get answers to your specific questions.